How To Redirect To Spring Security OAuth2 Behind a Gateway/Proxy – Part 1: Running Your App In Docker
Switching From Tutorial-Mode (aka POC) To Production Is Hard
Developing Your first OAuth2-App on localhost
with OAuth2 Boot may be easy, …
…but what about running it in real life?
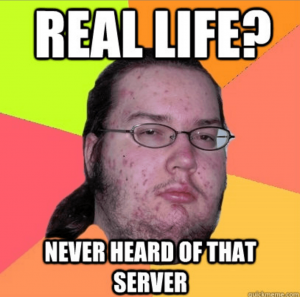
This is the first post of a series of Mini-Howtos, that gather some help, to get you started, when switching from localhost to production with SSL and a reverse-proxy (aka gateway) in front of your app, that forwards the requests to your app that listens on a different name/IP, port and protocol.
In This Series We Will…
- Start with the fantastic official OAuth2-Tutorial from the Spring-Boot folks – love it! – and run it as a container in docker
- Hide that behind a reverse-proxy, like in production – nginx in our case, but could be any pice of software, that can act as a gateway
- Show how to debug the oauth2-flow for the whole crap!
- Enable SSL for our gateway – because oauth2-providers (like Facebook) are pressing us to do so
- Show how to do the same with Facebook, instead of GitHub
I will also give some advice for those of you, who are new to Docker – but just enough to enable you to follow.
This is Part 1 of this series, that shows how to package a Spring-Boot-App as Docker-Image and run it as a container
tut-spring-boot-oauth2/logout
As an example for a simple app, that uses OAuth2 for authentication, we will use the third step of the Spring-Boot OAuth2-Tutorial.
You should work through that tutorial up until that step – called logout -, if you have not done yet. This will guide you through programming and setting up a simple app, that uses the GitHub-API to authenticate its users.
Especially, it explains, how to create and set up a OAuth2-App on GitHub – Do not miss out on that part: You need your own app-ID and -secret and a correctly configured redirect URI.
You should be able to build the app as JAR and start that with the ID/secret of your GitHub-App without changing code or configuration-files as follows:
mvn package
java -jar target/social-logout-0.0.1-SNAPSHOT.jar \
--spring.security.oauth2.client.registration.github.client-id=YOUR_GITHUB_APP_ID
--spring.security.oauth2.client.registration.github.client-secret=YOUR_GITHUB_APP_SECRET
If the app is running corectly, you should be able to Login/Logout via http://localhost:8080/
The folks at Spring-Boot are keeping the guide and this repository up-to-date pretty well. At the date of the writing of this article it is up to date with version 2.2.2.RELEASE of Spring-Boot.
You may as well use any other OAuth2-application here. For example your own POC, if you already have build one that works while running on localhost
Some Short Notes On OAuth2
I will only explain the protocol in very short words here, so that you can understand what goes wrong in case you stumble across one of the many pitfalls, when setting up oauth2. You can read more about oauth2 elswhere
For authentication, oauth2 redirects the browser of your user to a server of your oauth2-provider. This server authenticates the user and redirects the browser back to your server, providing additionally information and ressources, that lets your server know that the user was authenticated successfully and enables it to request more information in the name of the user.
Hence, when configuring oath2 one have to:
- Provide the URI of the server of your oauth2-provider, the browser will be redirected to for authentication
- Tell the server of the oauth2-provider the URL, the browser will be redirected to back after authentication
- Also, your app has to provide some identification – a client-ID and -secret – when redirecting to the server of your oauth2-provider, which it has to know
There are a lot more things, which can be configured in oauth2, because the protocol is designed to fit a wide range of use-cases. But in our case, it usually boils down to the parameters mentioned above.
Considering our combination of spring-security-oauth2
with GitHub this means:
- The redirect-URIs of well known oauth2-providers like GitHub are build into the library and do not have to be configured explicitly.
-
The URI, the provider has to redirect the browser back to after authenticating the user, is predefined by the library as well.
But as an additional security measure, almost every oauth2-provider requires you, to also specify this redirect-URI in the configuration on the side of the oauth2-provider.
This is a good and necessary protection against fraud, but at the same time the primary source for missconfiguration: If the specified URI in the configuration of your app and on the server of your oauth2-provider does not match, ALL WILL FAIL! - The ID and secret of the client (your GitHub-app) always have to be specified explicitly by hand.
Again, everything can be manually overriden, if needed.
Configuration-keys starting with spring.security.oauth2.client.registration.github
are choosing GitHub as the oauth2-provider and trigger a bunch of predifined default-configuration.
If you have set up your own oauth2-provider, you have to configure everything manually.
Running The App Inside Docker
To faciliate the debugging – and because this most probably will be the way you are deploying your app anyway – we will start by building a docker-image from the app
For this, you do not have to change a single character in the example project – all adjustments to the configuration will be done, when the image is started as a container.
Just change to the subdirectory logout
of the checked out project and create the following Dockerfile
there:
FROM openjdk:8-jre-buster
COPY target/social-logout-0.0.1-SNAPSHOT.jar /opt/app.jar
EXPOSE 8080
ENTRYPOINT [ "/usr/local/openjdk-8/bin/java", "-jar", "/opt/app.jar" ]
CMD []
This defines a docker-image, that will run the app.
- The image deduces from
openjdk:8-jre-buster
, which is an installation of the latest OpenJDK-JDK8 on a Debian-Buster - The app will listen on port
8080
- By default, a container instanciated from this image will automatically start the Java-app
- The
CMD []
overwrites the default from the parent-image with an empty list – this enables us to pass command-line parameters to our spring-boot app which we will need to pass in our configuration
You can build and tag this image with the following commands:
mvn clean package
docker build -t juplo/social-logout:0.0.1 .
This will tag your image as juplo/social-logout:0.0.1
– you obviously will/should use your own tag here, for example: myfancytag
Do not miss out on the flyspeck (.
) at the end of the last line!
You can run this new image with the follwing command – and you should do that, to test that everything works as expected:
docker run \
--rm \
-p 8080:8080 \
juplo/social-logout:0.0.1 \
--spring.security.oauth2.client.registration.github.client-id=YOUR_GITHUB_ID \
--spring.security.oauth2.client.registration.github.client-secret=YOUR_GITHUB_SECRET
--rm
removes this test-container automatically, once it is stopped again-p 8080:8080
redirects port8080
onlocalhost
to the app
Everything after the specification of the image (here: juplo/social-logout:0.0.1
) is handed as a command-line parameter to the started Spring-Boot app – That is, why we needed to declare CMD []
in our Dockerfile
We utilize this here to pass the ID and secret of your GitHub-app into the docker container — just like when we started the JAR directly
The app should behave exactly the same now lik in the test above, where we started it directly by calling the JAR.
That means, that you should still be able to login into and logout of your app, if you browse to http://localhost:8080
—
At least, if you correctly configured http://localhost:8080/login/oauth2/code/github
as authorization callback URL in the settings of your OAuth App on GitHub.
Comming Next…
In the next part of this series, we will hide the app behind a proxy and simulate that the setup is running on our real server example.com
.
Leave a Reply